Mastering Recursion in Kotlin: A Secret Weapon for Performance! 🚀
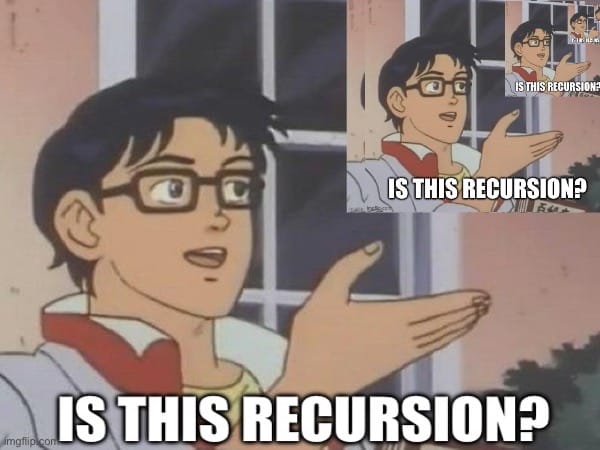
Ever hit a StackOverflowError while using recursion? 😨
Recursion often gets a bad reputation for being complex and inefficient. In traditional recursion, each function call adds a new frame to the stack, making deep recursion risky (hello, stack overflow! 😵).
Well, Kotlin has your back with tailrec!
Instead of deep recursive calls that risk stack overflow, tailrec optimizes recursion into a loop—making it memory-efficient & blazing fast. ⚡
//❌ Before: Regular Recursion (Risky for Large Inputs)
fun factorial(n: Int): Int {
return if (n == 0) 1 else n * factorial(n - 1) // 🚨 Stack grows!
}
😵 Issue? This function keeps stacking calls, leading to failure for large numbers like factorial(10000).
import java.math.BigInteger
// ✔ After: Tail-Recursive Optimization
tailrec fun factorialOptimized(n: Int, accumulator: BigInteger = BigInteger.ONE): BigInteger {
return if (n <= 1) accumulator else factorialOptimized(n - 1, accumulator * n.toBigInteger())
}
fun main() {
//println(factorial(10000))
println(factorialOptimized(50000)) // ✅ No StackOverflowError!
}
here is another example:
📌 Finding the Nth Fibonacci Number (Optimized with tailrec
)
🚀 Why tailrec
?
- Regular recursion in Fibonacci leads to exponential time complexity (
O(2^N)
) due to repeated calls. - Using
tailrec
eliminates redundant calls and runs in O(N) time complexity with no extra stack overhead.
❌ Before: Regular Recursive Fibonacci (Very Slow!)
fun fibonacci(n: Int): Int {
return if (n <= 1) n else fibonacci(n - 1) + fibonacci(n - 2) // 🚨 Too many redundant calls!
}
fun main() {
println(fibonacci(40)) // ❌ Very slow for large values
}
😵 Problem? Each call spawns two more calls, leading to exponential complexity!
✔ After: Tail-Recursive Fibonacci (Blazing Fast! 🚀)
tailrec fun fibonacciOptimized(n: Int, a: BigInteger = BigInteger.ZERO, b: BigInteger = BigInteger.ONE): BigInteger {
return if (n == 0) a else fibonacciOptimized(n - 1, b, a + b) // ✅ Tail call - no extra stack usage
}
fun main() {
println(fibonacciOptimized(10000)) // ✅ Works instantly, even for huge numbers!
}
✅ Why is tailrec
the best choice here?
- No exponential growth – Instead of
O(2^N)
, it runs in O(N) time! - No redundant calculations – Uses accumulators (
a, b
) to track previous values. - No stack overflow – Even
fibonacciOptimized(10000)
works instantly!
🎯 Why is tailrec a game-changer? When to Use tailrec
?
✅ When solving problems with high recursion depth (e.g., Fibonacci, factorial, tree traversal).
✅ Prevents Stack Overflow – No deep recursive calls!
✅ When an iterative approach is less readable but tail recursion is more elegant.
✅ Optimized by Kotlin Compiler – Converts recursion into a loop!
✅ More Efficient Execution – Faster & safer for large numbers!
Next time you use recursion, be cool 😎 and add tailrec where applicable!🔥 tailrec
is a hidden Kotlin superpower—use it wisely!
👉 Have you used tailrec before? Share your thoughts! 🚀 #Kotlin #Programming #Recursion