Jetpack Compose vs Compose Multiplatform: A Deep Dive From an Android Developer's Perspective
Comprehensive comparison of Jetpack Compose and Compose Multiplatform frameworks. Learn key differences, use cases, and implementation strategies for modern Android development.
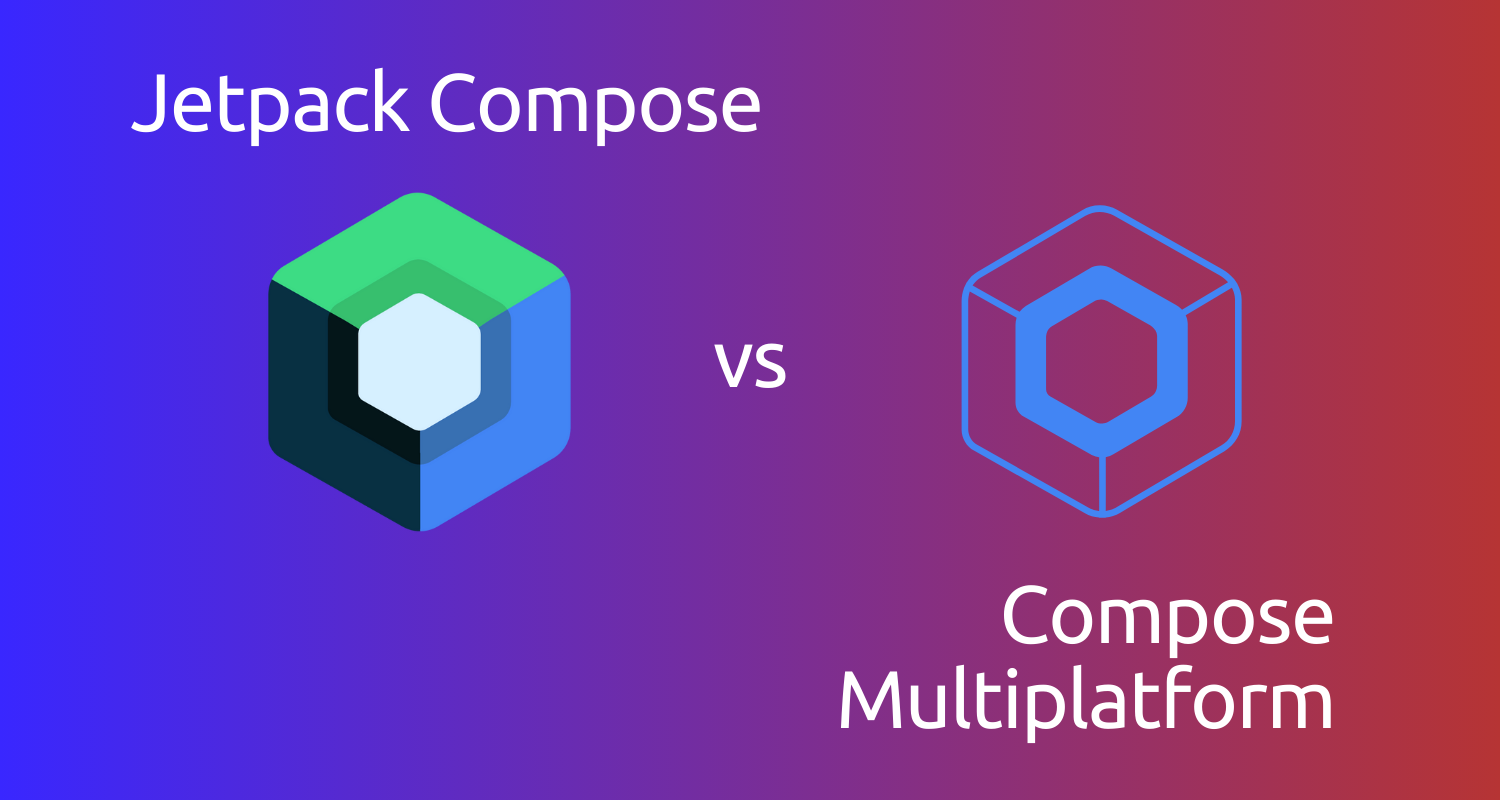
After working with both Jetpack Compose for several years and on Compose Multiplatform from Last One Year , I've gained valuable insights into their strengths, differences, and real-world applications. Let me take you through a comprehensive exploration of these technologies.
The Evolution of UI Development in Android
When I started Android development five years ago, XML layouts were the standard. We'd spend hours wrestling with ConstraintLayouts and handling configuration changes. Then came Jetpack Compose in 2021, revolutionizing how we build UIs in Android. More recently, Compose Multiplatform has expanded this paradigm beyond Android, offering a unified approach to UI development across platforms.
Understanding Jetpack Compose
Jetpack Compose is Google's modern toolkit for building native Android UIs. At its core, it brings declarative UI programming to Android, similar to how React works for web development. Instead of manually manipulating UI elements, we describe what we want, and Compose handles the rest.
Here's a simple example from one of my recent projects:
@Composable
fun ProductCard(
product: Product,
onProductClick: (Product) -> Unit
) {
Card(
modifier = Modifier
.fillMaxWidth()
.padding(8.dp)
.clickable { onProductClick(product) }
) {
Column(
modifier = Modifier.padding(16.dp)
) {
Text(
text = product.name,
style = MaterialTheme.typography.headlineSmall
)
Spacer(modifier = Modifier.height(8.dp))
Text(
text = product.description,
style = MaterialTheme.typography.bodyMedium
)
}
}
}
The beauty of this approach is its composability. Each UI element is a function that can be combined with others to create complex interfaces. The state management is explicit and predictable, making it easier to debug and maintain.
Enter Compose Multiplatform
Compose Multiplatform takes the core concepts of Jetpack Compose and extends them to other platforms. It's not just a wrapper around Jetpack Compose – it's a complete reimagining of how we can share UI code across platforms.
Here's how we can write shared UI code that works on both Android and iOS:
expect fun getPlatformName(): String
@Composable
fun SharedScreen() {
var counter by remember { mutableStateOf(0) }
Column(
modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally
) {
Text("Running on ${getPlatformName()}")
Button(onClick = { counter++ }) {
Text("Clicked $counter times")
}
}
}
// Platform-specific implementation for Android
actual fun getPlatformName(): String = "Android"
// Platform-specific implementation for iOS
actual fun getPlatformName(): String = "iOS"
The Deep Technical Differences
Now, let's dive into the more advanced aspects that I've encountered in production environments:
1. Threading Model
Jetpack Compose is tightly integrated with Android's threading model. It works seamlessly with Coroutines and the Android lifecycle. In contrast, Compose Multiplatform needs to abstract these platform-specific concerns. It uses an expect/actual pattern to handle threading across platforms:
// Shared code
expect fun runOnMainThread(block: () -> Unit)
// Android implementation
actual fun runOnMainThread(block: () -> Unit) {
MainScope().launch { block() }
}
// iOS implementation
actual fun runOnMainThread(block: () -> Unit) {
dispatch_async(dispatch_get_main_queue()) { block() }
}
2. Memory Management
One of the most challenging aspects I've dealt with is memory management. Jetpack Compose benefits from Android's garbage collection, but when working with Compose Multiplatform, especially on iOS, we need to be more careful about memory leaks:
class SharedViewModel {
private val scope = CoroutineScope(SupervisorJob() + Dispatchers.Main)
// This needs careful handling in iOS
private val stateFlow = MutableStateFlow<UiState>(UiState.Initial)
protected fun onCleared() {
scope.cancel() // Important for iOS memory management
}
}
3. Platform-Specific Features
While Compose Multiplatform aims for code sharing, there are times when we need platform-specific implementations. I've found that using the expect/actual pattern with interfaces works well:
interface ImageLoader {
suspend fun loadImage(url: String): ImageBitmap
}
expect fun createPlatformImageLoader(): ImageLoader
// Android implementation using Coil
actual fun createPlatformImageLoader(): ImageLoader = object : ImageLoader {
override suspend fun loadImage(url: String): ImageBitmap {
return Coil.execute(ImageRequest.Builder(context)
.data(url)
.build())
.drawable
.toBitmap()
.asImageBitmap()
}
}
Real-World Considerations
After working on several production apps, here are some key insights:
- Jetpack Compose is more mature for Android-specific features. If you're building an Android-only app with complex platform integrations, it might be the better choice.
- Compose Multiplatform shines in projects where you need to share significant UI code between platforms. However, be prepared for more setup complexity and potential platform-specific workarounds.
- The testing story is different for each. Jetpack Compose has excellent testing support through the Android testing libraries, while Compose Multiplatform requires more setup for cross-platform testing.
Looking Forward
The ecosystem is evolving rapidly. With the recent introduction of the Kotlin Multiplatform Mobile plugin and improving tooling support, Compose Multiplatform is becoming increasingly viable for production applications.
However, it's crucial to understand that choosing between Jetpack Compose and Compose Multiplatform isn't just a technical decision – it's a strategic one that depends on your team's expertise, project requirements, and long-term goals.